How to read Node.js environment variables
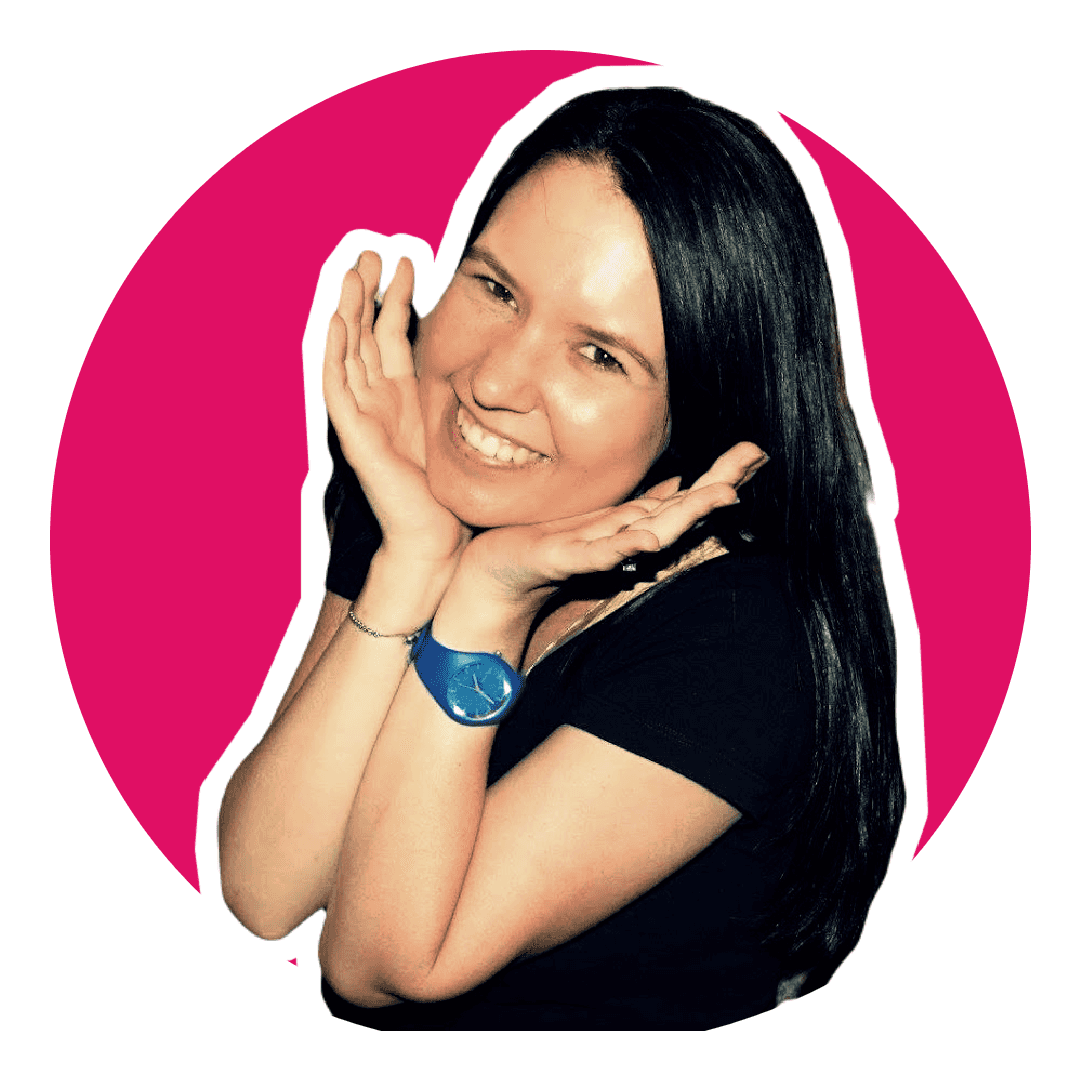
Software Engineering Team Lead and Director of Cloudsure
Running a Node app is simple.
node panic.js
Sometimes you want to add environment variables that either contain sensitive information or are contextual to the environment you want to run your app on. You can set them the moment the process is started.
NUMBER=42 ANIMAL=dolphins PREFIX=don\'t node panic.js
Imagine having to do this approach with multiple vars and for production ready systems where commands are written to the logs. Not a good idea. This approach is great for testing though.
To access these variables in your code, simply prefix the scream case with process.env.
.
console.log(`${process.env.NUMBER} is my favorite number.`);
console.log(`${process.env.ANIMAL} are my favourite animals`);
console.log(`${process.env.PREFIX} panic!`);
What you do then is create a hidden environment file in the root of your project most commonly named .env
.
NUMBER="42"
ANIMAL="dolphins"
PREFIX="don't"
If you have multiple environments, you can use dotenv to load them during runtime.
require('dotenv').config();
console.log(`${process.env.NUMBER} is my favorite number.`);
console.log(`${process.env.ANIMAL} are my favourite animals`);
console.log(`${process.env.PREFIX} panic!`);
Note
You don't need to import the
dotenv
Node package into your codebase. Instead run the command below to run the code and do a module to preload (option can be repeated) to require the dependency(ies).
node --require dotenv/config panic.js
# or shortened to
node -r dotenv/config panic.js
If you have multiple files for different environments then you can specify the
file by appending dotenv_config_path
to it.
node -r dotenv/config panic.js dotenv_config_path=.env-vogsphere
Append dotenv_config_debug=true
to the command to print out the values in the file.