Create a new React web application
React is currently claimed to be the most popular JavaScript library out there. In this chapter, you will learn more about getting started with React, create your React application and the basic pages and components required for this course.
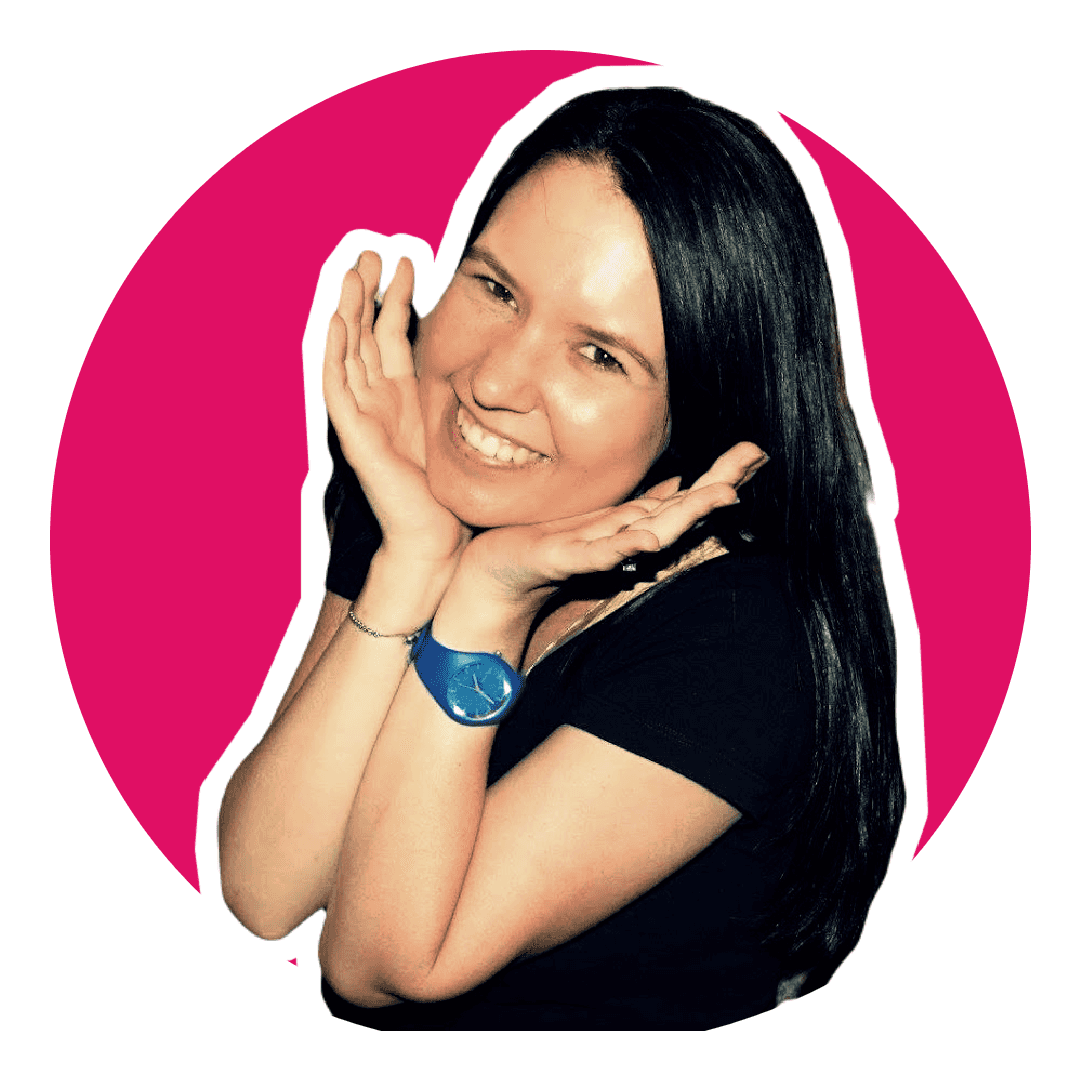
Software Engineering Team Lead and Director of Cloudsure
Objectives
- Learn some React.
- Create your React application.
- Create the basic pages and components required for this course.
Get started
React is currently claimed to be the most popular JavaScript library out there. Here's a video that will teach you more about it. What you’ll learn in the video below:
- How to use React
- Why you would want to use React
- How to start building React apps
Create your application
You can either use CRA or create the application from the ground up.
According to Facebook, CRA was not created for production but rather created for beginners so that developers didn't have to learn React and Webpack at the same time. CRA lacks flexibility as it is hard to configure, customize and strip out unnecessary dependencies.
I've called the application is stargazers but you can call it anything you want. The madness behind the name is that there will be stars on the website for rating a company review.
npx create-react-app stargazers
cd stargazers
Git repository
Don't forget to init your Git repository so that you can push to your favorite hosted Git service like GitHub, GitLab or BitBucket. Keep on committing during the course.
git init
git add .
git commit -m "Initial commit"
Verify
You should see a slow spinning React logo when the page loads on http://localhost:3000 when you start the app.
bashnpm start
Skeleton
Create your directory structure so that you have pages and components to work on.
mkdir src/pages && touch src/pages/Home.js src/pages/Register.js src/pages/Login.js src/pages/Review.js src/pages/NotFound.js
mkdir src/components && touch src/components/Layout.js
More pages and components to come later on.
Layout
Edit your Layout
component with some skeleton markup.
const Layout = ({ children }) => {
return <div>{children}</div>;
};
export default Layout;
Pages
Edit your page components to look like the page below but update the component name and children inside the Layout component to
reflect the name of the page you are editing. Example HomePage
and Home page
become LoginPage
and Login page
respectively.
const HomePage = () => {
return <div>Home page</div>;
};
export default HomePage;
App
Edit the component that mounts into your application.
Import the Layout
component and use it with some dummy text for the time being.
import Layout from './components/Layout';
function App() {
return <Layout>Something great is about to happen!</Layout>;
}
export default App;
Next steps
Now that you have the basic components and pages, you can routes to each page with a navigation component for users to click on.
References
- How to create a React app without using create-react-app - Dev.to
- Getting Started with React - DigitalOcean on YouTube