Get started
You will be exposed to various terminology, get started with the setup of services required for this course and create your own web application from scratch using React.
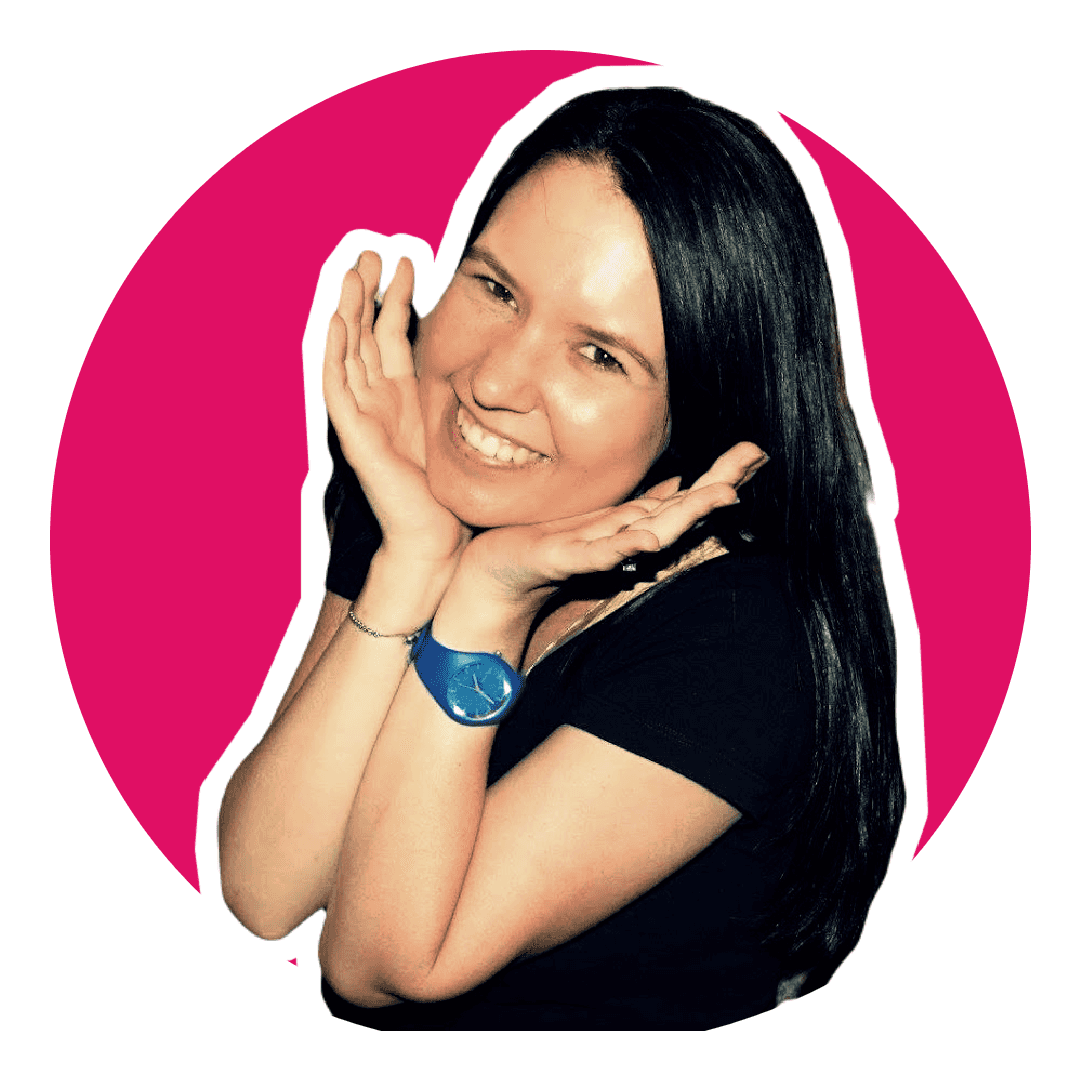
Software Engineering Team Lead and Director of Cloudsure
Objectives
- Cover what GitHub, Jamstack, Astra DB and Netlify are.
- Create a web application from scratch using React.
- Initialize Git so that you can push to a remote repository so that Netlify can auto deploy.
- Configure secret environment variables so that you can connect to your Astra DB database.
- Install a bunch of important dependencies required by your app.
- Install and configure the Netlify CLI.
π‘ I use the
tree
command to show files and directories inside a particular directory. Find out more here.
GitHub
According to GitHub, GitHub is a place to share code with friends, co-workers, classmates, and complete strangers. Millions of people use GitHub to build things together.
Jamstack
Jamstack is a web experience architecture where the experience layer and data/business logic layers are decoupled. The idea is to improve flexibility, scalability, performance, and maintainability.
Astra DB
Astra DB is a serverless, multi-cloud native Database as a Service provided by DataStax. It is built on Apache Cassandra and is meant to simplify application development and remove the need to self-host and manage a Cassandra database.
Setup your Astra DB
- Sign up or login to your DataStax Astra account.
- Create an Astra DB Database or use an existing one.
- Create an Astra DB Keyspace called
sag_todos
in your database. You can call your namespace anything really, just update references later on in the tutorial. - Generate an Application Token with the role of
API Admin User
for the Organization that your Astra DB is in. Download this file or store the details for later use as you will not be able to access it again.
Create a new project
Create an web app
You're going in quick and dirty using Create React App (CRA). Don't feel limited to using React. You could use any framework here. I chose this route because it was the fastest for me at the time.
Note about CRA π€
You're using CRA only because it is quick and simple to get up and running. According to Facebook, CRA was not created for production but rather created for beginners so that developers didn't have to learn React and Webpack at the same time. CRA lacks flexibility as it is hard to configure, customize and strip out unnecessary dependencies. To create a prod-ready version of your app with React then try this tutorial.
npx create-react-app my-primitive-todo-app
cd my-primitive-todo-app
Make a Git repository
π‘ If you are new to Git, check out my Git course to get started.
In a nutshell, you need to initialize Git, add and commit all your initial files, and connect and push to your GitHub (or other Git repository service that integrates with Netlify) repository.
git init
git add . # don't do this unless everything can be committed in one contextual go
git commit -m "Initial commit"
git remote add origin git@github.com:<your-username>/my-primitive-todo-app.git
git push -u origin main
β― tree -L 1 -la
.
βββ .git
βββ .gitignore
βββ README.md
βββ node_modules
βββ package-lock.json
βββ package.json
βββ public
βββ src
4 directories, 4 files
Environment variables
Create an environment variable file to put your Astra DB connection settings in.
touch .env .env.example
ASTRA_DB_ID=<ID>
ASTRA_DB_REGION=<REGION>
ASTRA_DB_APPLICATION_TOKEN=<TOKEN>
ASTRA_DB_KEYSPACE=<KEYSPACE>
Exclude this file from Git because it contains sensitive information that you most definitely don't want in your Git history or on public display.
.env
Put a copy of this template without it's values into an example file so that you can commit it and refer to it at a later stage.
ASTRA_DB_ID=
ASTRA_DB_REGION=
ASTRA_DB_APPLICATION_TOKEN=
ASTRA_DB_KEYSPACE=
Project dependencies
Install some dependencies. You can choose to use Yarn if you want to.
npm install --save-dev netlify-cli
npm install --save @astrajs/collections classnames uuid
- netlify-cli: interacts with Netlify from the command line interface.
- @astrajs/collections: connects your NodeJS app to your DataStax Astra DB or your Stargate instance.
- classnames: conditionally join class names together.
- uuid: for the creation of RFC4122 UUIDs.
{
"name": "my-primitive-todo-app",
"version": "0.1.0",
"private": true,
"dependencies": {
"@astrajs/collections": "^0.3.4",
"@testing-library/jest-dom": "^5.16.5",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"classnames": "^2.3.2",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"uuid": "^9.0.0",
"web-vitals": "^2.1.4"
},
"scripts": {
"start": "react-scripts start",
"build": "react-scripts build",
"test": "react-scripts test",
"eject": "react-scripts eject"
},
"eslintConfig": {
"extends": [
"react-app",
"react-app/jest"
]
},
"browserslist": {
"production": [
">0.2%",
"not dead",
"not op_mini all"
],
"development": [
"last 1 chrome version",
"last 1 firefox version",
"last 1 safari version"
]
},
"devDependencies": {
"netlify-cli": "^12.0.11"
}
}
Netlify
Get started with Netlify. Your development environment prerequisites are explained in depth their tutorial.
Command Line Interface
Install the Netlify CLI (lets you configure continuous deployment from the command line). You can use it to run a local development server, run local build and plugins and deploy your site.
Global versus local
Installing Netlify CLI globally means that your system always has the latest version, including any breaking changes. While global installation is appropriate for initial development and experimentation, for managing builds in a continuous integration (CI) environment, use local CLI installation instead.
npm install netlify-cli -g
You can configure continuous deployment for a new or existing site.
To create a new site without continuous deployment, use netlify sites:create
.
You will probably need to Authenticate to Netlify first.
netlify init
Go through the prompts to initialize Netlify.
Adding local .netlify folder to .gitignore file...
? What would you like to do? (Use arrow keys)
β Connect this directory to an existing Netlify site
β― + Create & configure a new site
? Team: (Use arrow keys)
β― Steve the hedge's team
? Site name (leave blank for a random name; you can change it later):
Site Created
Admin URL: https://app.netlify.com/sites/musical-cuchufli-95efd3
URL: https://musical-cuchufli-95efd3.netlify.app
Site ID: 6aa72498-9801-472c-ba69-24681d7f36de
Linked to musical-cuchufli-95efd3
? Your build command (hugo build/yarn run build/etc): react-scripts build
? Directory to deploy (blank for current dir): build
? No netlify.toml detected. Would you like to create one with these build settings? Yes
Adding deploy key to repository...
Deploy key added!
Creating Netlify GitHub Notification Hooks...
Netlify Notification Hooks configured!
Success! Netlify CI/CD Configured!
This site is now configured to automatically deploy from github branches & pull requests
Next steps:
git push Push to your git repository to trigger new site builds
netlify open Open the Netlify admin URL of your site
Alternatively, you can link a local repo or project folder to an existing site on Netlify.
bashnetlify link
Authenticate and make sure your site is linked to a Netlify siteID
.
Once it has been configured your site will automatically deploy from GitHub branches and pull requests.
git push # Push to your git repository to trigger new site builds
netlify open # Open the Netlify admin URL of your site
Add the Netlify dev script to your package.json
file. This will run the netlify server
and host your localhost site on port 8888
by default.
"scripts": {
"dev": "netlify dev",
}
Netlify installed and configured a bunch of things on your behalf.
[build]
command = "react-scripts build"
functions = "netlify/functions"
publish = "build"
The local Netlify folder was ignored from Git.
.netlify
Start your application using the npm script below and see the default CRA Learn React rotating atom screen. \o/
npm run dev
β Server will be ready on http://localhost:8888
Environment variables on Netlify
- Go to your site on Netlify
- Click on the Site Overview tab
- Click on the Site settings buttons
- Click on the Build & deploy navigation item
- Click on the Environment sub navigation item
- Click on Edit variables button
- Add the variables in your environment variable file to Netlify
Clean up
Your app is full of stuff you don't need. Let's delete some stuff and create new stuff.
rm -rf ./src
mkdir src
cd src
touch api.js index.js App.js
Mount the app
You need to mount your app into the root
element in your index HTML file.
import React from 'react';
import ReactDOM from 'react-dom/client';
import App from './App';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<React.StrictMode>
<App />
</React.StrictMode>
);
You can quickly update the page title and manifest files while you are at it.
<title>My Awesome Task List</title>
π« Deploy
Push to GitHub, login to Netlify, and verify the build of your site. If all went well, run it and party π
Conclusion
You have a working skeleton React app that is automatically deployed to the cloud using Netlify. You are ready to write your API that will integrate with Astra DB.
Your project should finally look like this.
β― tree -L 1 -la
.
βββ .env
βββ .env.example
βββ .git
βββ .gitignore
βββ .netlify
βββ README.md
βββ netlify
βββ netlify.toml
βββ node_modules
βββ package-lock.json
βββ package.json
βββ public
βββ src
6 directories, 7 files
β Remember to commit your files to Git and then push in order to have Netlify build and deploy your app.
References
- DataStax Todo Astra Jamstack Netlify Example - GitHub
- Jamstack - Official website
- DataStax - Official website
- Netlify - Official website